Overview
FlashSpeed is a text-based flashcard application specifically designed for university students who are learning a foreign language.
Users are able to categorize virtually created flashcards into their own decks and launch review sessions within those decks.
Summary of contributions
My roles in this project include being in charge of the weekly agenda, minutes taker and in-charge of the Developer Guide. Being in charge of the weekly agenda meant that I briefed team members of the upcoming tasks to be discussed every meeting. I also took down any important discussion points needed to complete to agenda items. I also established deadlines for weekly tasks.
-
Major enhancement: Added the game algorithm for the game mode.
-
What it does:
-
In a game, the next card is always randomly chosen.
-
If the card is answered incorrectly, the user will have to answer that card another time.
-
However, the user only needs to answer correctly 2 times in a row to clear that card. For example, if the user gets the same card wrong 10 times in a row, he only needs to answer that card 2 times to clear it.
-
If the user answers the card correctly one time and wrong after that. The user will still need to answer the card correctly twice in a row.
-
-
If the card is answered correctly on the first try, that card is cleared and the user no longer has to answer it.
-
-
Justification: This feature improves the product significantly because it helps the user memorize words more effectively as well as making the app more challenging and fun to use. Users can memorize words more effectively when the ones they get wrong are shown more often.
-
Highlights: This algorithm affected existing features, such as the Statistics at the end of the game as well as the progress bar of the game. It required the team to decide how we should re-implement the existing affected features to accommodate this enhancement.
-
-
Other major enhancement: Added the Card and its relevant classes.
-
What it does: Just like a traditional flashcard, a Card allows users to enter content for the front and back faces of a Card.
-
Justification: This feature is essential to FlashSpeed. Without it, there is no flashcard representation in our app.
-
Highlights: This enhancement required very in-depth analysis of design alternatives, such as where should we implement it, how should it interact with upper classes (Model, UI, Storage, Deck). It required a few hours of discussion and debate before we finally settled on the final design.
-
Credits: AB3 Person Class - The skeleton of the Card class was derived from that Class.
-
-
Minor enhancement: Re-implemented how some parsers handled user input by using Java Regex. This was implemented to simplify how we got parameters especially for the shortcut formats that we wanted to implement for EditCardCommand.
-
Minor enhancement: Re-implemented the Help Window to display the entire User Guide instead of just the link.
-
Code contributed: [Functional code] [Test code]
-
> 5000 LoC
-
Note: Although there is a huge difference in LOC shown on tP Dashboard, my team contributed very evenly to functional code.
-
-
Other contributions:
-
Project management:
-
Setting up Github and Travis
-
Setting up and maintaining the Milestone Tracker
-
Maintaining most of the issues on issue tracker
-
-
Enhancements to existing features:
-
Documentation contributed:
-
Developer Guide:
-
In-charge of document quality
-
Reformatted the structure and layout for ease of readability and navigability (e.g. topic headings, section spacing, etc.)
-
Wrote introduction
-
Wrote the entire Appendix A-G
-
-
Others:
-
Review/mentoring contributions:
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Renaming a deck : rename
Format: rename <index> <deck>
You can rename a deck in the library using rename
followed by the index number of the deck and the new name of the deck.
The deck name cannot by empty. |
Examples:
-
rename 2 Japanese Verbs
Renames the 2nd deck in the library to "Japanese Verbs".
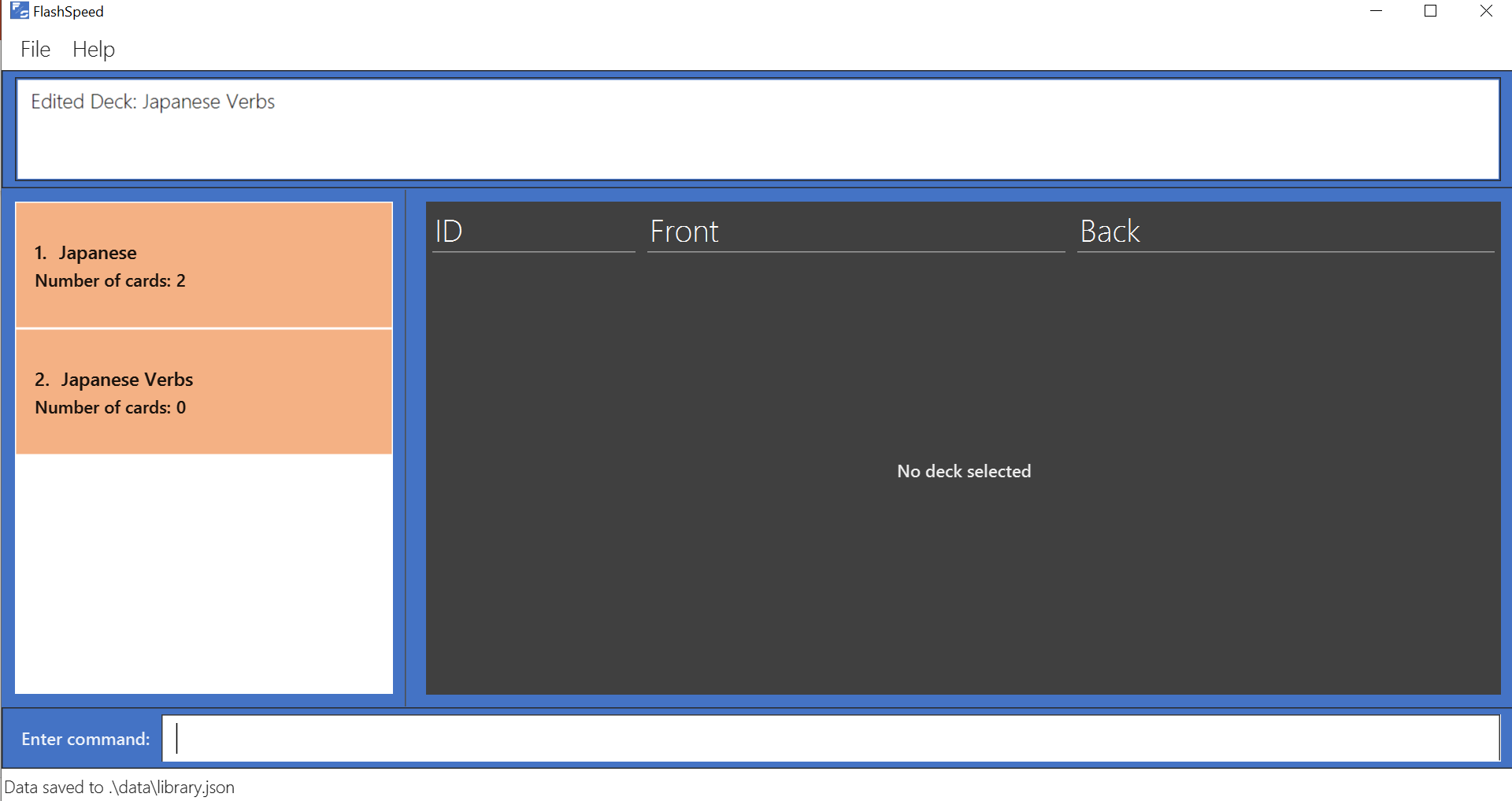
Playing a deck : play
Format: play <index>
Alright, this is what you’ve been waiting for! In order to start a study session with a certain deck,
type play
followed by the deck’s index number and press Enter. Good luck on your learning journey.
FYI: we "play" a deck because learning is fun!
Example:
-
play 1
Starts a study session with the 1st deck in the library.
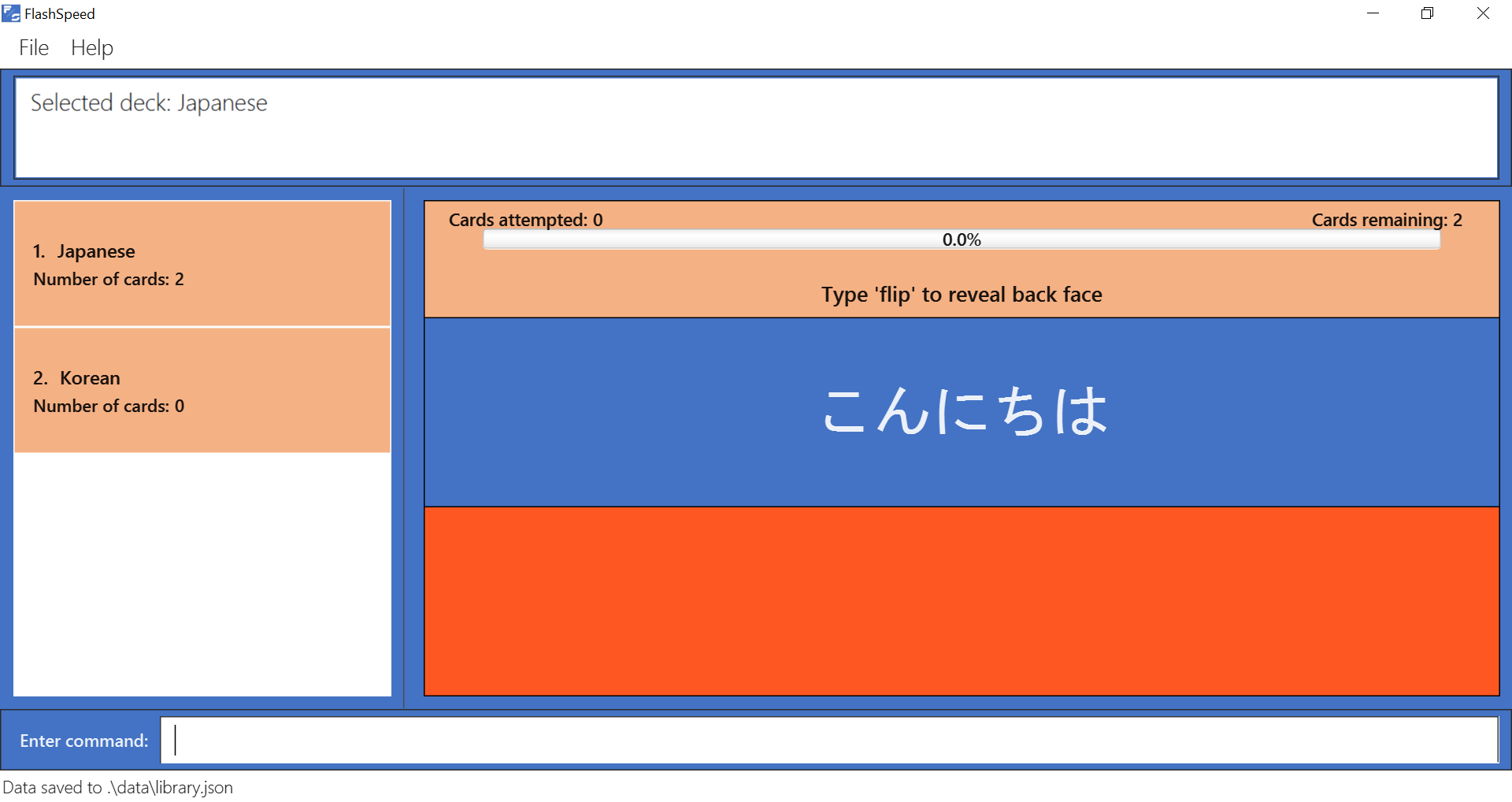
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Introduction
FlashSpeed is a text-based flashcard application specifically designed for university students who are learning a foreign language.
FlashSpeed enhances the revision process by implementing a smarter review system in its design. Users will be tested more frequently on words that they have gotten wrong in a game session.
The main features of FlashSpeed allows users to:
Purpose
This document specifies architecture, software design decisions and implementation for the FlashSpeed application.
Audience
This document is intended for anyone who wants to understand the system architecture, design and implementation of FlashSpeed.
The following groups are in particular are the intended audience of this document.
-
FlashSpeed developers
-
FlashSpeed features enhancement team members
How to use
-
Section 3 - Design contains information about the main components of FlashSpeed.
-
Firstly, refer to 3.1 Architecture section to learn more about the overall architecture.
-
Then proceed to sections 3.2 - 3.6 to learn more about each individual component in the architecture.
-
-
Section 4 - Implementation contains information about the main commands and the implementations of each command used in FlashSpeed.
-
Appendix - Information related to the development process and design choices of FlashSpeed.
Logic component
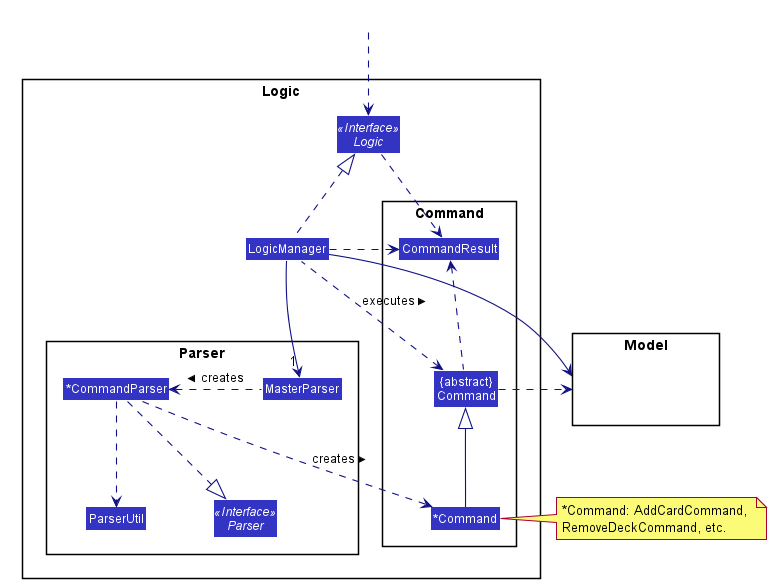
API :
Logic.java
-
Logic
uses theMasterParser
class to parse the user command. -
This results in a
Command
object which is executed by theLogicManager
. -
The command execution can affect the
Model
(e.g. adding a card). -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
. -
In addition, the
CommandResult
object can also instruct theUi
to perform certain actions, such as displaying help to the user.
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("delete 1")
API call.
Design Considerations
Aspect: If the user is already viewing another deck and decides to create a new deck, there was a consideration whether to switch the UI for the user view to the new deck or continue to let the user view the current deck.
-
Alternative 1 (current choice): Switch the view to the new Deck
-
Pros: Able to use the new Deck immediately without typing an additional command to select it.
-
-
Alternative 2: Keep the view at the current Deck
-
Pros: Don’t have to type in an additional command to return back to the current Deck if a new Deck is created
-
We chose Alternative 1 in the end as we believed that it will be more likely for the user to want to use the new deck immediately after creating it.
Design Considerations
Aspect: Using yes
and no
instead of the actual answer.
-
Alternative 1 (current choice): Using a simple yes or no
-
Pros: User can definitively choose if their answer was correct or not. This leads to accurate evaluation and statistics calculation.
-
Cons: Not as interactive as if the user were to type in the correct word/sentence itself.
-
-
Alternative 2: Typing in the actual answer itself.
-
Pros: More interactive to the user.
-
Cons: Typos or slightly incomplete (but correct) answers can be typed it by the user. As the answers typed in mush exactly match the one on the card, it may result in inaccurate evaluation and statistics calculation at the end of the game.
-
UC03: Delete a deck
MSS:
-
FlashSpeed shows a list of decks.
-
User chooses a deck and deletes it.
-
The deck disappears from the list of decks.
Use case ends.
Extensions
-
1. The list is empty.
Use case ends.
-
2a. The given index is invalid.
-
2a1. FlashSpeed shows an error message.
Use case resumes at step 1.
-
UC04: View a deck (select)
MSS:
-
FlashSpeed shows a list of all decks.
-
Uer chooses a deck and requests to view that deck.
-
FlashSpeed shows a list of all cards in the deck.
Use case ends.
Extensions
-
1. The list is empty.
Use case ends.
-
2a. The given index is invalid.
-
2a1. FlashSpeed shows an error message.
Use case resumes at step 1.
-
{more at Appendix C in DG}
Appendix A: Glossary
- Mainstream OS
-
Windows, Linux, Unix, macOS.
- Deck
-
A Deck is a group of Cards.
- Card
-
A Card mimics a physical flashcard. It has two faces. One side for prompting the user and the other side for the content the user wants to memorize.
- Smarter Review System
-
The smarter review system is adopted from the well known Space Repetition System. Cards that are answered wrongly in Play Mode will be shown more frequently in this system.
- Space Repetition System
-
Spaced repetition is an evidence-based learning technique that is usually performed with flashcards. Newly introduced and more difficult flashcards are shown more frequently while older and less difficult flashcards are shown less frequently in order to exploit the psychological spacing effect.
{Appendix naming is not correct here, more at Appendix E in DG}
Deck functionality
-
Creating a deck.
-
Prerequisites: None
-
Test case:
create Russian
Expected: Deck shows up on the deck list on the left panel and the (currently empty) card list is shown on the right panel. -
Test case:
create x
(where x is a deck name that already exists)
Expected: No new deck is created. Error details shown in the status message. Status bar remains the same. -
Other incorrect create commands to try:
create
, `create ` Expected: Similar to previous
-
-
Deleting a deck from the deck list shown on the left panel.
-
Prerequisites: Ensure that FlashSpeed contains at least 1 Deck, which can be seen on the left panel.
-
Test case:
remove 1
Expected: First deck is deleted from the list. Name of the deleted deck is shown in the status message. Timestamp in the status bar is updated. -
Test case:
remove 0
Expected: No deck is deleted. Error details shown in the status message. Status bar remains the same. -
Other incorrect delete commands to try:
remove
,remove x
(where x is larger than the list size)
Expected: Similar to previous.
-
-
Renaming a deck.
-
Prerequisites: Ensure that FlashSpeed contains at least 1 Deck, which can be seen on the left panel.
-
Test case:
rename 1 Russian
Expected: New deck name shows up on the deck list on the left panel. -
Test case:
rename 0 Russian
Expected: No deck is renamed. Error details shown in the status message. Status bar remains the same. -
Other incorrect create commands to try:
rename Russian
,rename
,rename x Russian
(where x is larger than the list size),rename 1 y
(where y is a deck name that already exists)
Expected: Similar to previous.
-
Card functionality
-
Adding a card to a deck.
-
Prerequisites: A deck needs to be selected first via
select INDEX
-
Test case:
add 아녕하세요 : hello
Expected: Card shows up on the card list on the right panel. -
Test case:
add 안녕:하세요:hello
Expected: No card is created. Error details shown in the status message. Status bar remains the same. -
Other incorrect create commands to try:
add 안녕하세요: `, `add :hello
Expected: Similar to previous
-
-
Deleting a card from the card list shown on the right panel.
-
Prerequisites: A deck needs to be selected first via
select INDEX
-
Test case:
delete 1
Expected: First card is deleted from the list. Name of the deleted card is shown in the status message. Timestamp in the status bar is updated. -
Test case:
delete 0
Expected: No card is deleted. Error details shown in the status message. Status bar remains the same. -
Other incorrect delete commands to try:
delete
,delete x
(where x is larger than the list size)
Expected: Similar to previous.
-
-
Editing a card.
-
Prerequisites: A deck needs to be selected first via
select INDEX
-
Test case:
edit 1 안녕 : Hi!
Expected: New card information is reflected on the card list on the left panel. -
Test case:
edit 1 안녕:
Expected: New card information (the front side) is reflected on the card list on the left panel. -
Test case:
edit 1 : Hi!
Expected: New card information (the back side) is reflected on the card list on the left panel. -
Test case:
edit 0 안녕하세요: Hi!
Expected: No card is edited. Error details shown in the status message. Status bar remains the same. -
Other incorrect create commands to try:
edit
,edit test : test test
,edit x Russian : 러시안어
(where x is larger than the list size),rename 1 y
(where y is a card that already exists)
Expected: Similar to previous.
-
{more at Appendix G in DG}